Topic – Creating Custom Record Fetcher Component with Salesforce lighting web components.
What you will learn here
- how to get list of all custom and standard object in LWC.
- get all fields of selected object in LWC.
- how to call apex method from lightning component .
- how to use lightning-checkbox-group
Final Outcome
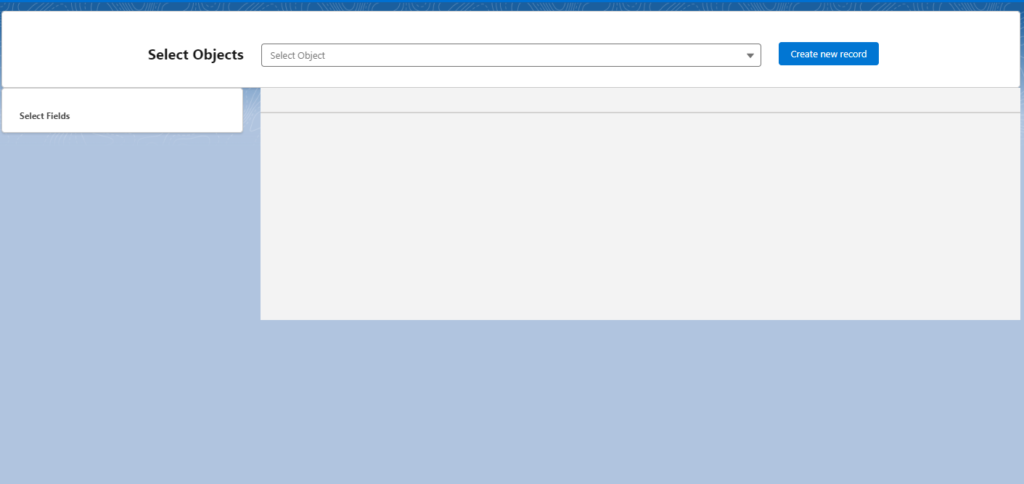
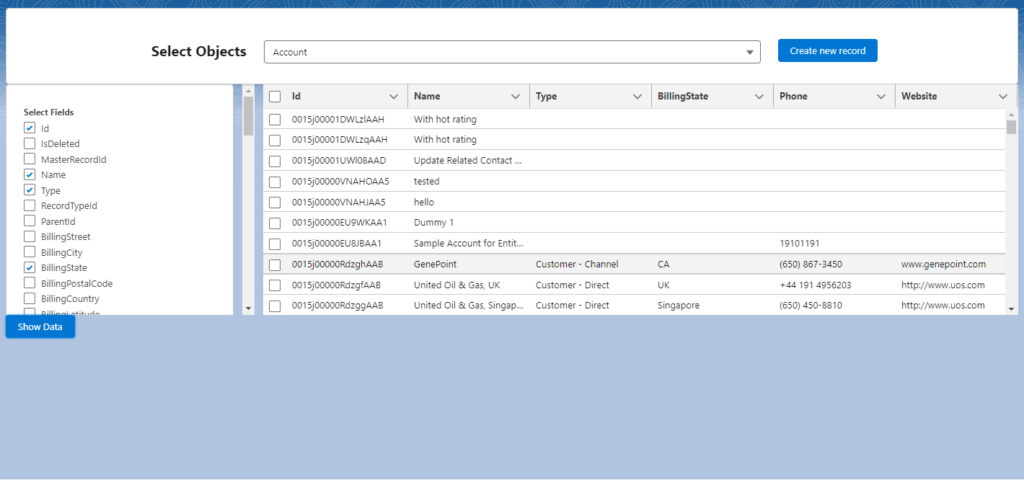
html
<template>
<lightning-card >
<div class="slds-grid slds-gutters slds-grid_vertical-align-center">
<div class=" slds-col slds-size_3-of-12 " style="text-align:right">
<h1 class="slds-text-heading_medium "><b>Select Objects</b></h1>
</div>
<div class="slds-col slds-size_6-of-12 slds-col slds-float_left slds-m-bottom_medium ">
<lightning-combobox
name="ObjectList"
onchange={onObjectChange}
options={objectList}
placeholder="Select Object">
</lightning-combobox>
</div>
<div class="slds-col slds-col slds-size_4-of-12 ">
<button class="slds-button slds-button_brand cancel-or-save-or-update-button" onclick={handleOpenModel} disabled={disableUpdateBtn}>
<label>Create new record</label>
</button>
</div>
</div>
</lightning-card>
<div class="slds-grid slds-gutters">
<div class="slds-col slds-size_3-of-12 slds-scrollable_y " style="height:20rem;">
<lightning-card >
<div class="slds-card">
<lightning-checkbox-group class="slds-m-left_large"
name="SelectFields"
label="Select Fields"
options={lstFields}
value = {lstFields}
onchange={handleCheckBoxClick}>
</lightning-checkbox-group>
</div>
</lightning-card>
</div>
<div class="slds-col slds-size_9-of-12 slds-scrollable_y" style="height:20rem;" >
<lightning-datatable
key-field="id"
columns={columnsMap}
data = {accountList}>
</lightning-datatable>
</div>
</div>
<div if:true={showButton}>
<lightning-button label="Show Data" name="ShowData" variant="Brand" style="margin-top: 15px;" onclick={handleShowData}></lightning-button>
</div>
<template if:true={openModel}>
<section role="dialog" tabindex="-1" aria-labelledby="modal-heading-01" aria-modal="true" class="slds-modal slds-fade-in-open">
<lightning-spinner alternative-text="Loading" size="small" if:true={showSpinner}></lightning-spinner>
<div class="slds-modal__container">
<div class="slds-modal__header">
<h1 class="slds-modal__title slds-hyphenate">Create new {objectName}</h1>
</div>
<div class="slds-modal__content slds-var-p-around_large">
<lightning-record-edit-form
object-api-name={objectName}>
<template for:each={columnsMap} for:item="field">
<lightning-input-field
key={field.label}
field-name={field.label}
onchange={handleFieldValueChange}>
</lightning-input-field>
</template>
</lightning-record-edit-form>
<div class="slds-text-align_right">
<button class="slds-button slds-button_neutral cancel-or-save-or-update-button" aria-label="Cancel and close" onclick={closeModel}>
<label>Cancel</label>
</button>
<button class="slds-button slds-button_brand cancel-or-save-or-update-button" onclick={createRecord} disabled={disableUpdateBtn}>
<label>Save</label>
</button>
</div>
</div>
</div>
</section>
<div class="slds-backdrop slds-backdrop_open" role="presentation"></div>
</template>
</template>
js
import { LightningElement } from 'lwc';
import {api , track , wire } from 'lwc'
import fetchAllObjectList from '@salesforce/apex/RecordFetcherController.fetchAllObjectList';
import fetchgetAllFields from '@salesforce/apex/RecordFetcherController.fetchgetAllFields'
import fetchAllRecordsOfSelectedObject from '@salesforce/apex/RecordFetcherController.fetchAllRecordsOfSelectedObject'
export default class MyRecordFetcher extends LightningElement {
@track lstFields = [];
@track objectList = [];
objectName = '';
showButton = false;
arrayToSend = [];
accountList = [];
arrayToSend = [];
columnsMap=[];
openModel = false;
connectedCallback() {
fetchAllObjectList()
.then((result) => {
if (result) {
this.objectList = [];
for (let key in result ) {
this.objectList.push({ label: key, value: key });
}
} else {
console.log('Objects are not found')
}
}).catch((error) => {
console.log('Objects are not found')
});
}
onObjectChange(event) {
this.lstFields = [];
this.accountList = [];
this.columnsMap=[];
this.lab=[];
this.val=[];
this.arrayToSend=[];
this.objectName = event.detail.value;
this.showButton = true
this.getFieldsHandle();
}
getFieldsHandle(){
fetchgetAllFields({ strObjectName: this.objectName })
.then(result => {
this.lstFields = [];
for (let key in result) {
this.lstFields.push({ label: key, value: key });
}
})
.catch(error => {
console.log('All fields are not fetched');
})
}
handleGetRecordsOfSelectObject() {
fetchAllRecordsOfSelectedObject({ strObjectName: this.objectName })
.then(result => {
this.accountList = result;
})
.catch(error => {
console.log('error while getting records ' , error);
})
}
handleCheckBoxClick(event) { // getting proxy in event.detail.value
this.arrayToSend = [];
for(let index in event.detail.value) {
this.arrayToSend.push(event.detail.value[index])
}
var val = this.arrayToSend;// same ['Id','Name']
console.log('-->',val);
this.columnsMap = val.map((v,i) =>
({label: val[i], fieldName:v})
)
console.log('this.columnsMap',val);
}
handleShowData() {
this.handleGetRecordsOfSelectObject();
}
handleOpenModel() {
this.openModel = true;
}
closeModel() {
this.openModel = false;
}
handleFieldValueChange(event) {
console.log('field value : ' + event.detail.value);
console.log('field name : ' + event.target.fieldName);
}
}
apex
public with sharing class RecordFetcherController {
@AuraEnabled(cacheable = true)
public static Map<String,String> fetchAllObjectList(){
Map<String,String> mapOfAllObject = new Map<String,String>();
for(Schema.SObjectType objTyp : Schema.getGlobalDescribe().Values())
{
String name = objTyp.getDescribe().getLocalName();
String label = objTyp.getDescribe().getLabel();
mapOfAllObject.put(name,label);
}
System.debug('mapOfAllObject' + mapOfAllObject);
return mapOfAllObject;
}
@AuraEnabled(cacheable=true)
public static Map<String,String> fetchgetAllFields(String strObjectName) {
Map<String, Schema.SObjectType> detail = Schema.getGlobalDescribe();
Map<String,String> mapOfFields = new Map<String,String>();
for(Schema.SObjectField fields :detail.get(strObjectName).getDescribe().fields.getMap().Values()) {
mapOfFields.put(fields.getDescribe().getName() , fields.getDescribe().getName());
}
return mapOfFields;
}
@AuraEnabled(cacheable=true)
public static List<sObject> fetchAllRecordsOfSelectedObject(String strObjectName) {
System.debug(' strObjectName '+ strObjectName);
Map<String, Schema.SObjectType> detail = Schema.getGlobalDescribe();
List<String> allFieldsOfSelectedObject = new List<String>();
for(Schema.SObjectField fields :detail.get(strObjectName).getDescribe().fields.getMap().Values()) {
allFieldsOfSelectedObject.add(fields.getDescribe().getName());
}
string allstring = string.join(allFieldsOfSelectedObject,',');
String query = 'select ' + allstring +' from ' + strObjectName;
// query+= ' limit 10';
System.debug(' Database quqey '+ Database.query(query));
return Database.query(query);
}
}
Share your quesitons/feedback.
Thank you.