Woo woo…. welcome back dear coders !
Let’s build something fresh today . If you are new to the lightning world then this small LWC project will take your LWC and Salesforce knowledge one level up.
Today we are going to build ToDo app in salesforce with the help of lightning components . Only Prerequisite get started with these tutorial is – you must have VS code setup for salesforce development.
Here is the brief summary of steps that we are going to follow to build our ToDo app project :
Summary :
- Create new salesforce app named as Todo
- Create custom object named as ToDo
- create APEX class ToDoController
- Create LWC names as todoapp
- create a tab for our LWC in our Lightning app
For the developers who are too hurry and want to see how final output will be ,here is the snap of todo app that we are going to build.
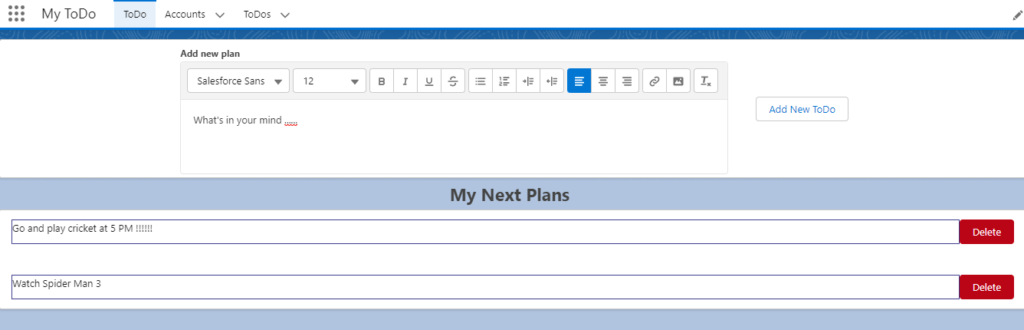
Now let’s move and start the implementation –
Step 1 : Create the new lighting app
Go to your salesforce org , in the quick find box search for app manager and create a new Lighting app.

Step 2 – Create new salesforce Object
Now we have to create the Custom Object which will holds the structure of our ToDo app , To create a new object in salesforce find object manager and create new object named as ToDo .

once you have the object go to Fields & Relationships and create fields for ToDo Name to hold the Name of ToDo and ToDoRecord to save actual ToDo record.
Watch the video where we will build this ToDo app together , this video will walk you through each step in detail .
Step 3 : Create APEX class ToDoController
We need to create on APEX class which will have methods to create new ToDo record , delete selected ToDo record and method to list out available ToDo records .
Class ToDoController.cls
public with sharing class ToDoController {
@AuraEnabled
public static List<ToDo__c> saveToDo(String doDoName , String record){
try {
ToDo__c myToDo = new ToDo__c();
String shortName = doDoName.substring(0,5);
myToDo.Name = shortName;
myToDo.ToDoRecord__c = record;
Database.insert(myToDo);
System.debug([SELECT Id, ToDoRecord__c, Name FROM ToDo__c]);
return [SELECT Id, ToDoRecord__c, Name FROM ToDo__c ORDER BY createdDate DESC];
} catch (Exception e) {
throw new AuraHandledException(e.getMessage());
}
}
@AuraEnabled
public static List<ToDo__c> getToDos() {
return [SELECT Id, ToDoRecord__c, Name FROM ToDo__c ORDER BY createdDate DESC];
}
@AuraEnabled
public static List<ToDo__c> deleteTodo(String toDoId){
try {
List<ToDo__c> toDoItem = [SELECT Id from ToDo__c WHERE Id =: toDoId];
delete toDoItem;
return [SELECT Id, ToDoRecord__c, Name FROM ToDo__c ORDER BY createdDate DESC];
} catch (Exception e) {
throw new AuraHandledException(e.getMessage());
}
}
}
Step 4 : Create Lightning Component
Now we have object , APEX class with methods to create and delete ToDo’s , now go to your VS Code and create new lightning component , inside your HTML file add the given code .
todoapp.html
<template >
<div class="slds-card slds-grid slds-grid_vertical slds-align_absolute-center">
<div class="slds-col slds-grid">
<div class="slds-m-around_xx-small slds-align_absolute-center">
<div class="slds-form-element">
<b style="height: 30px;"><label class="slds-form-element__label" for="text-input-id-47">Add new plan</label></b>
<div class="slds-form-element__control">
<lightning-input-rich-text value="" data-id={index} onchange={getToDo} > </lightning-input-rich-text>
</div>
</div>
</div>
<div class="slds-m-left_x-large slds-align_absolute-center ">
<lightning-button onclick={addToDo} label="Add New ToDo"></lightning-button>
</div>
</div>
</div>
<div class="slds-align_absolute-center">
<b ><label style="font-size: 23px;" class="slds-form-element__label" for="text-input-id-47">My Next Plans</label></b>
</div>
<div class="slds-card slds-grid slds-grid_vertical">
<template for:each={accountList} for:item="lineItem">
<article class="slds-card" key={lineItem.key}>
<div class="slds-card__header slds-grid">
<div class="slds-media__body" style="border: 1px solid rgb(59, 59, 141);">
{lineItem.ToDoRecord__c}
</div>
<div class="slds-no-flex">
<button class="slds-button slds-button_destructive" onclick={deleteTodo} value={lineItem.Id}>Delete</button>
</div>
</div>
</article>
</template>
</div>
</template>
In our HTML file we have button for adding ToDo which onclick method names as addToDo and we have another button for delete ToDo with deleteTodo method. We also have lightning-input-rich-text on which we have onchange event where we will prepare our final toDo record value .
In our JavaScript file first import all required methods .
todoapp.js
import { LightningElement, track, wire} from 'lwc';
import saveToDo from "@salesforce/apex/ToDoController.saveToDo";
import getToDos from "@salesforce/apex/ToDoController.getToDos";
import deleteTodo from "@salesforce/apex/ToDoController.deleteTodo";
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
export default class Todoapp extends LightningElement {
@track todoRecord;
@track columns = [
{ label: 'ToDoRecord__c', fieldName: 'ToDoRecord__c' },
{ label: 'Id', fieldName: 'Id'}
];
@track accountList;
connectedCallback() {
getToDos()
.then((data) => {
this.accountList = data;
})
.catch((error) => {
this.toToastMessage('Error In Getting ToDo Items',JSON.stringify(error),'error');
});
}
getToDo(event) {
this.todoRecord = event.target.value ;
}
addToDo() {
this.todoRecord = this.todoRecord.replaceAll('</p>','')
this.todoRecord = this.todoRecord.replaceAll('<p>','')
this.todoRecord = this.todoRecord.replaceAll('<br>','')
this.accountList = [];
this.createToDoRecord();
}
createToDoRecord() {
saveToDo({ doDoName: this.todoRecord, record: this.todoRecord })
.then((data) => {
this.accountList = data;
this.toToastMessage('Success','ToDo Created','success');
})
.catch((error) => {
this.toToastMessage('Error in create todo',JSON.stringify(error),'error');
});
this.getAllToDos();
}
deleteTodo(event) {
this.accountList = [];
let toDoToDelete = event.target.value;
deleteTodo({ toDoId: toDoToDelete })
.then((data) => {
this.accountList = data;
this.toToastMessage('Success','ToDo deleted successfully','warning');
})
.catch((error) => {
this.toToastMessage('Error In delete',JSON.stringify(error),'error');
});
this.getAllToDos();
}
toToastMessage(title, message, variant) {
this.dispatchEvent(new ShowToastEvent({ title: title, message: message, variant: variant }));
}
}
Hey awasome …. well done …. Congrats on creating your first lighting project . If you have any concern then feel free to share with us .
Thank You ………!!!!