Suppose that you want to get record types of any standard or custom object, here is the simplest way to solve your problem.
First you have to import uiObjectInfoApi in your component js file , uiObjectInfoApi is an multipurpose method/class which helps you fetch object information , which include object field , record types and other information related to selected object. You just need to know how to use that properly .
I have created two record types on contact object and master will be the default record .
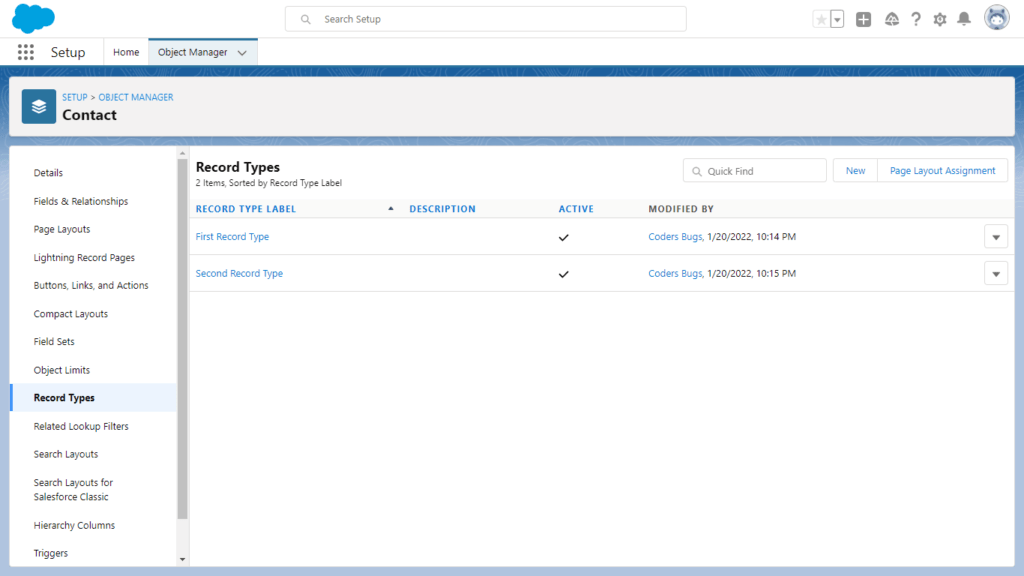
Now lets see how to get record types of contact object and use as a options of combobox.
import { LightningElement,api,track,wire } from 'lwc';
import { getObjectInfo } from 'lightning/uiObjectInfoApi';
export default class GetFieldsOfObjects extends LightningElement {
@track objectNameToGetRecordTypes = 'Contact';
@track lstRecordTypes =[];
@wire(getObjectInfo, { objectApiName: '$objectNameToGetRecordTypes' })
getObjectInfo({ error, data }) {
if (data) {
this.lstRecordTypes = [];
for (let key in data.recordTypeInfos) {
this.lstRecordTypes.push({ value: key, label:data.recordTypeInfos[key].name});
}
}
else if (error) {
console.log('Error while get record types');
this.lstRecordTypes = [];
}
}
}
Above code will give list of record types available on contact object, now lets use that list in our combobox.
<div class="slds-card">
<lightning-combobox name="fieldList" label='Field List' options={lstRecordTypes} placeholder="Select Field"></lightning-combobox>
</div>
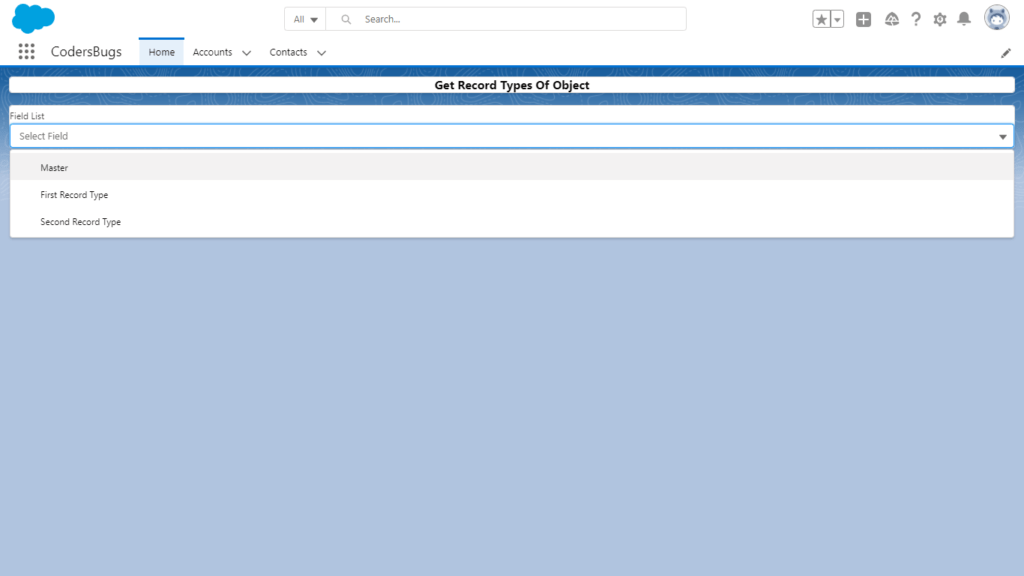
These is how simple we can get record types from any object and use those in our lwc component.