This blog shares the information about how to get all picklist or multi-picklist type fields from any given standard or custom salesforce object and on selection of picklist field how to fetch available picklist options.
Look into the given quick GIF to figure out how our final output will be look like.
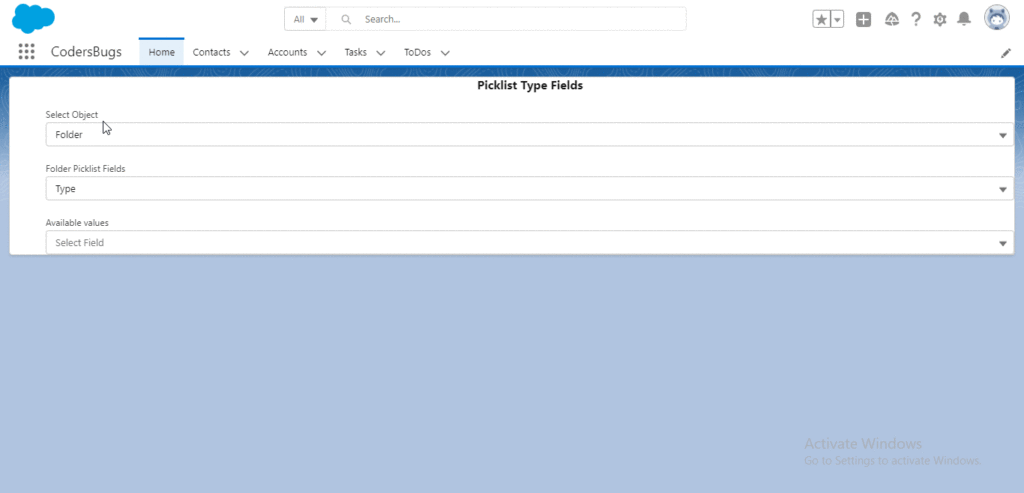
For the final result we will have three lightling-combobox , 1 – Select Object ,2 – Select Field and 3 – for Available options .
Let’s start from collecting all object list , we will call APEX method in LWC connectedCallback which will provide all standard and custom objects list to our first combobox . Use the following method to get all salesforce object .
Method to get all objects available in salesforce org.
@AuraEnabled(cacheable = true)
public static Map<String,String> getAllObjectList(){
Map<String,String> mapOfAllObject = new Map<String,String>();
for(Schema.SObjectType objTyp : Schema.getGlobalDescribe().Values())
{
Schema.DescribeSObjectResult describeSObjectResultObj = objTyp.getDescribe();
String name = objTyp.getDescribe().getLocalName();
String label = objTyp.getDescribe().getLabel();
mapOfAllObject.put(name,label);
}
return mapOfAllObject;
}
In the connectedCallback call getAllObjectList method
connectedCallback() {
getAllObjectList()
.then((result) => {
if (result) {
this.objectList = [];
for (let key in result ) {
this.objectList.push({ label: key, value: key });
}
} else {
console.log('Error in getting objects ')
}
}).catch((error) => {
console.log('Catch Error in getting objects ')
});
}
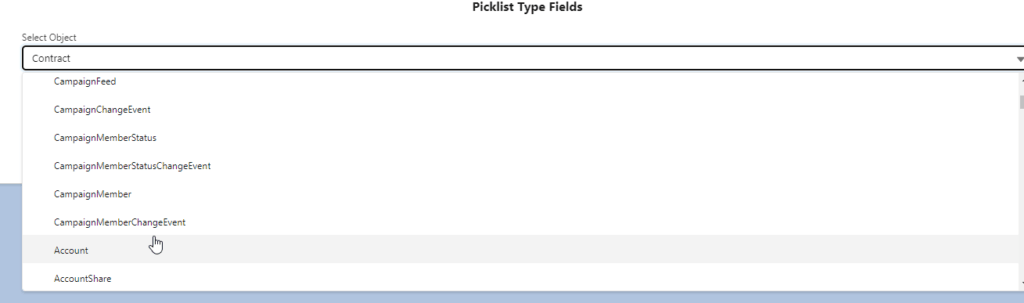
Once you have object list , let’s move on getting pick-list type fields for any selected object . For that we have following method which will take object name as parameter and return map of available pick-list type fields.
Method to get pick-list type fields from object
@AuraEnabled(cacheable=true)
public static Map<String,String> getPicklistTypeFields(String strObjectName) {
Map<String, Schema.SObjectType> detail = Schema.getGlobalDescribe();
Map<String,String> mapOfPicklistTypeFields = new Map<String,String>();
for(Schema.SObjectField fields :detail.get(strObjectName).getDescribe().fields.getMap().Values()) {
If(fields.getDescribe().getType() == Schema.DisplayType.PICKLIST) {
mapOfPicklistTypeFields.put(fields.getDescribe().getName() , fields.getDescribe().getName());
}
}
return mapOfPicklistTypeFields;
}
Note : When you want to send data from LWC to APEX method the parameter names must be same on both sides.
Now on object change we have to call getPicklistTypeFields APEX method which will take selected object as parameter and return lstOfPicklistFields available on given object.
onObjectChange(event) {
this.objectName = event.detail.value;
this.picklistFieldsLabel = '';
this.picklistFieldsLabel = this.objectName + ' Picklist Fields';
this.handleGetPicklistFields();
}
handleGetPicklistFields(){
getPicklistTypeFields({ strAccountName: this.objectName })
.then(result => {
this.lstOfPicklistFields = [];
for (let key in result) {
this.lstOfPicklistFields.push({ label: key, value: key });
}
})
.catch(error => {
console.log('Error in getting picklist fields');
})
}
Suppose that you have selected the account object then you will get following result
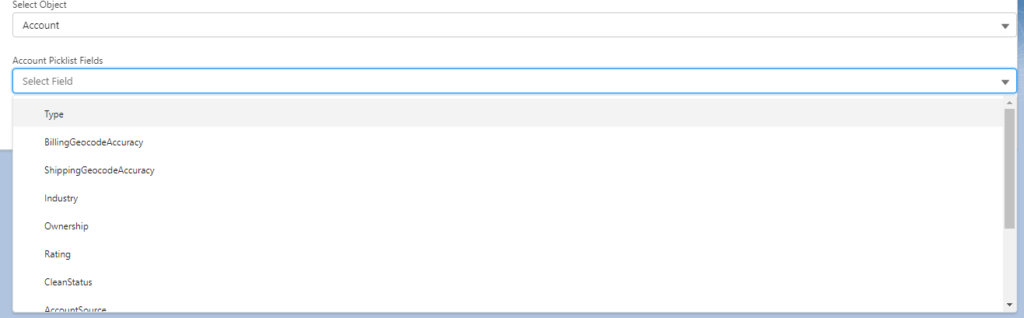
Now in some of the cases you may have have a requirement where you want get available options for selected picklist field , that is also easy . Let’s see how to achieve that
Here is the method to get picklist options for selected picklist field .
@AuraEnabled(cacheable = true)
public static Map<String,String> getOptionsForSelectedPicklistField(string selectedObjectName, string selectedField){
try {
System.debug('selectedObjectName '+selectedObjectName);
System.debug('selectedField '+selectedField);
Map<String,String> options = new Map<String,String>();
Map<String, Schema.SObjectField> mapFields = Schema.getGlobalDescribe().get(selectedObjectName).getDescribe().fields.getMap();
Schema.DescribeFieldResult pickFieldResult = mapFields.get(selectedField).getDescribe();
List<Schema.PicklistEntry> picklistFields1 = pickFieldResult.getPicklistValues();
for( Schema.PicklistEntry pickListFields2 : picklistFields1)
{
options.put(pickListFields2.getLabel(),pickListFields2.getValue());
}
return options;
} catch (Exception e) {
return null;
}
}
This method has a two parameter first is selectedObjectName and second is selectedField which should be a picklist or multi-picklist type field.
now let’s call this method into our lightning component .
getPicklistFieldsOptions(event) {
this.fieldSelectedToGetPicklistTypeField = event.detail.value;
this.getPicklistValuesForSelectedPicklistField();
}
async getPicklistValuesForSelectedPicklistField() {
await getPickListvaluesByFieldName({ objectName: this.objectName, pickListFieldName: this.fieldSelectedToGetPicklistTypeField })
.then((result) => {
if (result) {
this.objectFieldOptionsList = [];
for (let key in result) {
this.objectFieldOptionsList.push({ label: key, value: result[key] });
}
}
}).catch((error) => {
console.log('Error in getting pickist values')
})
}
This method will give you objectFieldOptionsList .
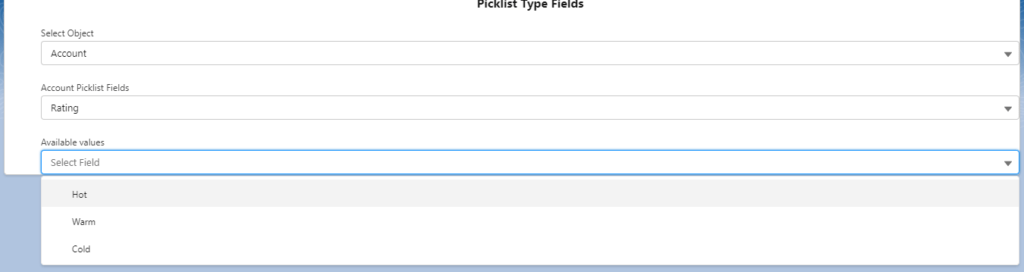
Let’s do a recap of what we have learn :
- How to get list of all objects from salesforce org .
- How to get all picklist / multi-picklist type fields of selected object .
- How to get picklist options for selected picklist type field .
Here is the youtube video where we have explained same concept step by step .
Here is the complete code for what we have done !
LWC HTML File code :
<template>
<div class="slds-card">
<h1 class="slds-card__header-title slds-align_absolute-center">Picklist Type Fields</h1>
</div>
<div class="slds-card">
<lightning-combobox name="ObjectList" label='Select Object' onchange={onObjectChange} options={objectList} placeholder="Select Object"></lightning-combobox>
</div>
<div class="slds-card">
<lightning-combobox name="fieldList" label={picklistFieldsLabel} onchange={getPicklistFieldsOptions} options={lstOfPicklistFields} placeholder="Select Field"></lightning-combobox>
</div>
<div class="slds-card">
<lightning-combobox name="fieldList" label='Available values' options={objectFieldOptionsList} placeholder="Select Field"></lightning-combobox>
</div>
</template>
LWC JS File code :
import { LightningElement } from 'lwc';
import {api , track , wire } from 'lwc'
import getPicklistTypeFields from '@salesforce/apex/GetPicklistTypeFields.getPicklistTypeFields'
import getAllObjectList from '@salesforce/apex/CommonMethods.getAllObjectList';
import getPickListvaluesByFieldName from '@salesforce/apex/GetPicklistTypeFields.getPickListvaluesByFieldName';
export default class GetPicklist extends LightningElement {
@track lstOfPicklistFields = [];
@track objectList = [];
@track mapofPickListValues = [];
@track objectFieldOptionsList = [];
@track picklistFieldsLabel = 'Picklist Fields'
fieldSelectedToGetPicklistTypeField = '';
objectName = '';
connectedCallback() {
getAllObjectList()
.then((result) => {
if (result) {
this.objectList = [];
for (let key in result ) {
this.objectList.push({ label: key, value: key });
}
} else {
console.log('Error in getting objects ')
}
}).catch((error) => {
console.log('Catch Error in getting objects ')
});
}
onObjectChange(event) {
this.objectName = event.detail.value;
this.picklistFieldsLabel = '';
this.picklistFieldsLabel = this.objectName + ' Picklist Fields';
this.handleGetPicklistFields();
}
handleGetPicklistFields(){
getPicklistTypeFields({ strAccountName: this.objectName })
.then(result => {
this.lstOfPicklistFields = [];
for (let key in result) {
this.lstOfPicklistFields.push({ label: key, value: key });
}
})
.catch(error => {
console.log('Error in getting picklist fields');
})
}
getPicklistFieldsOptions(event) {
this.fieldSelectedToGetPicklistTypeField = event.detail.value;
this.getPicklistValuesForSelectedPicklistField();
}
async getPicklistValuesForSelectedPicklistField() {
await getPickListvaluesByFieldName({ objectName: this.objectName, pickListFieldName: this.fieldSelectedToGetPicklistTypeField })
.then((result) => {
if (result) {
this.objectFieldOptionsList = [];
for (let key in result) {
this.objectFieldOptionsList.push({ label: key, value: result[key] });
}
}
}).catch((error) => {
console.log('Error in getting pickist values')
})
}
}
APEX class GetPicklistTypeFields :
public with sharing class GetPicklistTypeFields {
@AuraEnabled(cacheable=true)
public static Map<String,String> getAllPicklistTypeFields(String strAccountName) {
Map<String, Schema.SObjectType> detail = Schema.getGlobalDescribe();
Map<String,String> mapOfPicklistTypeFields = new Map<String,String>();
for(Schema.SObjectField fields :detail.get(strAccountName).getDescribe().fields.getMap().Values()) {
If(fields.getDescribe().getType() == Schema.DisplayType.PICKLIST) {
mapOfPicklistTypeFields.put(fields.getDescribe().getName() , fields.getDescribe().getName());
}
}
return mapOfPicklistTypeFields;
}
@AuraEnabled(cacheable = true)
public static Map<String,String> getOptionsForSelectedPicklistField(string selectedObjectName, string selectedField){
try {
System.debug('selectedObjectName '+selectedObjectName);
System.debug('selectedField '+selectedField);
Map<String,String> options = new Map<String,String>();
Map<String, Schema.SObjectField> mapFields = Schema.getGlobalDescribe().get(selectedObjectName).getDescribe().fields.getMap();
Schema.DescribeFieldResult pickFieldResult = mapFields.get(selectedField).getDescribe();
List<Schema.PicklistEntry> picklistFields1 = pickFieldResult.getPicklistValues();
for( Schema.PicklistEntry pickListFields2 : picklistFields1)
{
options.put(pickListFields2.getLabel(),pickListFields2.getValue());
}
return options;
} catch (Exception e) {
return null;
}
}
}
If you have question or concern the feel free to ask .
Thank you !!!!!
Very Helpful Video.
Thank You…
HI I have tried this code.. Very helpful.. I have a slightly different requirement.. I have a picklist field in the content version object with values New and Old.. I have edited all the file version details of the objects and given a value new or old for all the file’s versions. So i have to created a lwc folder in each object and for files with new picklist value I have to display them under the new folder and similarly for the old.. Can you help me on this
Can you please share more details about what you are trying to achieve?
Yes sure
My current situation is I have an object named FS where some files are uploaded. Also in the content version object I have a picklist Category with values “new” and “old”. So in each of the file versions I have an option to choose this picklist value as new or old. I have given the values for these files accordingly. For some file’s content versions picklist I have given new and the rest old. So my requirement is I have to display and lwc folder with two sections: New and Old, where new folder should contain all the attachments with the picklist value new and the old folder should contain all the attachments with picklist value old.
Right now I have just created an lwc component to display all the files uploaded in a particular record in the FS object. But I have to separate them as folders according to the earlier condition.
What if we want to fewtch state picklist field from address standard field?