In this tutorial we will show you real time example of sending data from child lwc component to parent lwc component.
suppose that you have an requirement where you have two lightning component Parent & Child , and you are using your child component into your parent lwc component .
Now you want to use data from child into parent , here we will give you simple way to solve this problem .
First you have to create child component where you can have number of input field , in my case I am using only on input field .
childC.html
<template>
<h1>I am Child</h1>
<lightning-input label="Input Box" name="inputbox" onchange={sendInputValuesToParent}></lightning-input>
</template>
childC.js
import { LightningElement } from 'lwc';
export default class ChildC extends LightningElement {
sendInputValuesToParent(event) {
this.dispatchEvent(
new CustomEvent("inputchange", { detail: { value: event.target.value, name: this.name } })
);
}
}
You can see we have have one input field which has onchange method ,sendInputValuesToParent method has event parameter which will help to to take input data .
To send data from this child we have to use dispatchEvent in our sendInputValuesToParent method which we be fire on changing the input .
Now let’s see how we make use of this event into our parent component.
parentC.html
<template>
<div class="slds-card">
<h1>I am Parent</h1>
<p> valueFromChild = {valueFromChild}</p>
</div>
<div class="slds-card">
<c-child-c oninputchange={handleValuesFromChild}></c-child-c>
</div>
</template>
parentC.js
import { LightningElement , track } from 'lwc';
export default class ParentC extends LightningElement {
@track valueFromChild='';
handleValuesFromChild(event) {
this.valueFromChild = event.detail.value;
}
}
Here in our parent component we have refer our child with oninputchange method which will be call to handleValuesFromChild method
NOTE : – We have to use prefix (on) when we refer any dispatch event from child into parent. In our case we have inputchange event in child that’s why we have refer as oninputchange in parent.
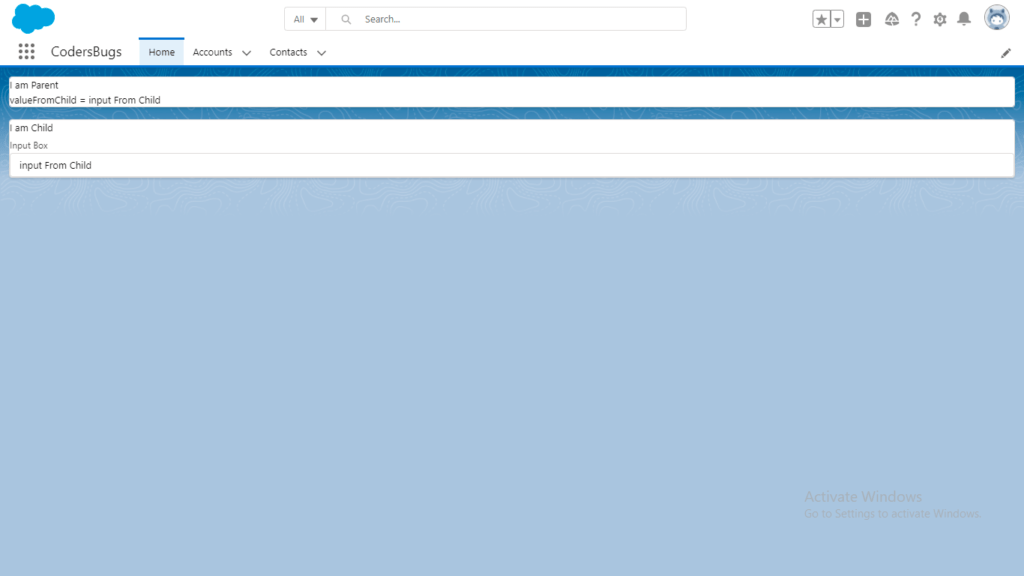
See the above image for final output .